withRouter
- HoC(Higer-order Component)
- 라우트로 사용된 컴포넌트가 아니어도 match, location, history 객체에 접근 가능
1. WithRouterSample.js
import React from "react";
import { withRouter } from "react-router-dom";
const WithRouterSample = ({ location, match, history }) => {
return (
<div>
<h4>location</h4>
<textarea
value={JSON.stringify(location, null, 2)}
rows={7}
readOnly={true}
/>
<h4>match</h4>
<textarea
value={JSON.stringify(match, null, 2)}
rows={7}
readOnly={true}
/>
<button onClick={() => history.push("/")}>Go Home</button>
</div>
);
};
export default withRouter(WithRouterSample);
export할 때, withRouter 함수로 감싸주기.
(JSON.stringify의 두 번째, 세 번째 파라미터를 null,2로 설정해주면
JSON에 들여쓰기가 적용된 상태로 문자열이 만들어진다.)
2. Profiles.js
import React from "react";
import { Link, Route } from "react-router-dom";
import Profile from "./Profile";
import WithRouterSample from "./WithRouterSample";
const Profiles = () => {
return (
<div>
<h3>User List</h3>
<ul>
<li>
<Link to="/profiles/yumcoding">yumcoding</Link>
</li>
<li>
<Link to="/profiles/myfav">MyFav</Link>
</li>
</ul>
<Route path="/profiles" exact render={() => <div>Choose an User</div>} />
<Route path="/profiles/:username" component={Profile} />
<WithRouterSample />
</div>
);
};
export default Profiles;
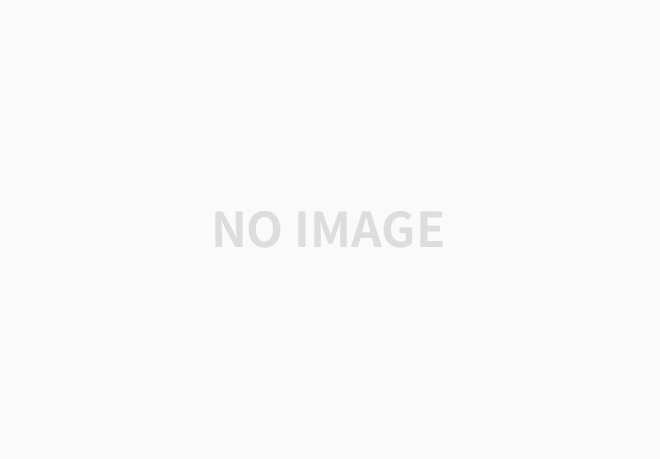
근데 match 객체의 params가 비어있음..
withRouter를 사용하면 현재 자신을 보여 주고 있는 라우트 컴포넌트(여기서는 Profiles)를 기준으로 match가 전달 됨.
Profiles를 위한 라우트를 설정할 때 path="/profiles"라고만 입력했기 때문에 username 파라미터를 읽어오지 못하는 것.
-> WithRouterSample 컴포넌트를 Profiles에서 지우고
Profile 컴포넌트에 넣어보기
import React from "react";
import {withRouter} from 'react-router-dom';
import WithRouterSample from './WithRouterSample';
const data = {
yumcoding: {
name: "yumyum",
description: "a developer who loves to play with React",
},
myfav: {
name: "serang",
description: "my favourite novelist",
},
};
const Profile = ({ match }) => {
const { username } = match.params;
const profile = data[username];
if (!profile) {
return <div>Not found</div>;
}
return (
<div>
<h3>
{username}({profile.name})
</h3>
<p>{profile.description}</p>
<WithRouterSample/>
</div>
);
};
export default withRouter(Profile);
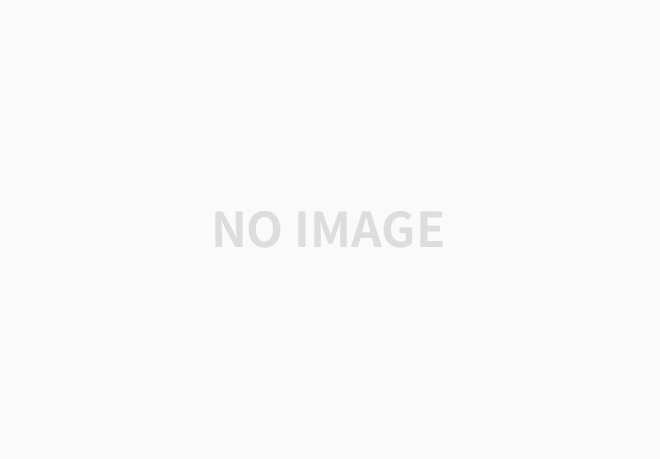
이제 match 객체의 params가 제대로 나오는 것을 확인할 수 있다.